Intro
In case you have worries that you might not implement everything correctly from the last lecture, you can check out to the branch called 1_server
. If you are good with your implementation, there is no need to check out to this branch.
This approach also applies to all the other lectures. You can either check out to the branch where you have all previously implemented code ready for you, or you can keep up working in the same branch.
The project structure should look like this:
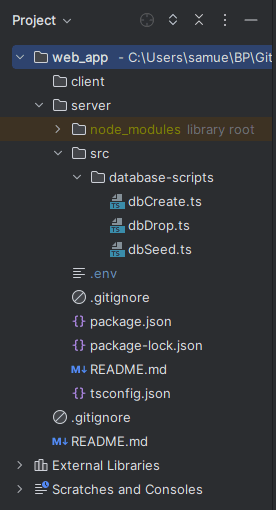
Server
For your app, we are going to use an Express.js server, which is a minimal and flexible Node.js web application framework.
- In the server`s src folder, create a file called
index.ts
. - Paste the following code to the file:
// Importing necessary modules
import express, {Application} from "express";
import cors from "cors";
import dotenv from "dotenv";
// Loading environment variables from .env file
dotenv.config();
// Creating an instance of the express application
const app: Application = express();
// Defining a default port for the server to listen to
const PORT = process.env.PORT || 5000;
// MIDDLEWARE
app.use(cors()); // Allow Cross-Origin Resource Sharing
app.use(express.json()); // Parse incoming request body as JSON
app.use(express.urlencoded({extended: true})); // Parse incoming request body as URL-encoded data
// ROUTES
// Starting the server and listening for incoming requests on the defined port
try {
app.listen(PORT, (): void => {
console.info(`Server is successfully running on port ${PORT}`);
});
} catch (error) {
console.error(`Error occurred: ${error.message}`);
}
Go through individual lines of code and try to understand their purpose.
- In line 13, we are defining a default port for the server to listen to in case the environment variable
PORT
is not defined.
We need to define the port in the environment variable file.
Edit the.env
file and add the following lines:
# Server configuration
PORT=5000
- In the
package.json
file, find the command which starts the server.
After running the command npm run dev
, the server should start and you should see the message Server is successfully running on port 5000
in the terminal. Also, the server is run using nodemon, which means that the server will restart automatically after any changes in the code.
Database
For the database, we are using PostgreSQL.
The database server is already running on the VM, so we are just going to create the database, tables and seed the tables with some data.
Before running following commands, terminate (CTRL + C) the running server in the terminal and insert the commands in the server directory
Create a database
In the terminal, run the following command:
npm run db-create
Create tables and insert data
Run the following command:
npm run db-seed
Drop database
If you corrupt the database and data in it during exposing the vulnerabilities, you can drop the database and create it again.
Do not run this command now, because it will erase everything you just created.
npm run db-drop
In case of need, to recreate the database, tables, and records, run npm run db-drop
, npm run db-create
and npm run db-seed
commands.
[Optional] Interact with the database
- To interact with the PostgreSQL database server, you can use
psql
CLI.
- You can type SQL commands to interact with the database, and it also provides meta-commands to interact with the server.
- Second option is to use preinstalled GUI tool called DBeaver, but we strongly recommend using
psql
CLI. A tutorial for these two tools is not part of this tutorial, but there are many available online.
Summary
By following the tutorial, you should have an Express server running on port 5000 and a database with seeded data. Let's move to the next section!